Servlet Interview questions and Answers
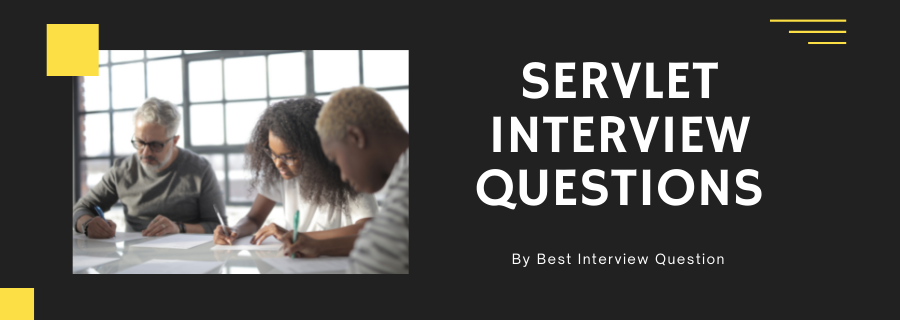
The Servlet is a class used in Java applications for enhancing the capabilities of the server. Java servlets run on web application server container and are used to generate HTML content that shows on the web browser. Servlets respond to request from the browser and return response content. Our new collection of Servlet interview questions is a must-read for all developers. Servlets are mostly implemented for HTTP protocol and are also called as HTTP Servlet.
About Servlet | |
---|---|
What is Servlet | Servlets generate dynamic HTML content and respond with excel, XML, pdf, and JSON and other formats. Initially, servlets were meant for creating HTML content in applications, but now they are mostly used at the controller layers. |
Latest Version | 4.0, released in September 2017. |
Created By | Pavni Diwanji |
Specification | JSR 369 |
Platform | Java EE 8 |
Important Changes | HTTP/2 |
Most Frequently Asked Servlet Interview questions
Servlet | CGI | |
---|---|---|
1. | Portable | Not portable |
2. | Requests handled by lightweight Java Thread | Requests handled by heavyweight OS process |
3. | Data sharing possible | Data sharing not available |
4. | Link directly to the Web server | Cannot link directly to the Web server |
Servlert’s life cycle is the entire process from its creation until its destruction. A Servlet follows this path:
- Initialized by calling init( ) method
- Calls service( ) method to process request
- Terminated by destroy( ) method
Finally, the servlet is nothing but the garbage that gets collected by JVM's garbage collector.
The Servlet is a class used in Java applications for enhancing the capabilities of the server. Servlets respond to a request from the browser and return response content and also run on web application server container and are used to generate HTML content that shows on the web browser.
There are two types of servlets - generic and HTTP
Generic Servlet | HTTP Servlet | |
---|---|---|
1. | Subclass of javax.servlet.GenericServlet | Subclass of javax.servlet.HttpServlet |
2. | Protocol independent | Protocol dependent |
3. | Belongs to javax.servlet package | Belongs to javax.servlet.http package |
GET method | POST method | |
---|---|---|
1. | A limited amount of data can be sent as data is sent in the header | Lot of data can be sent as data is sent in the body |
2. | Not secured because data is exposed | Secured because data is not exposed |
3. | Can be bookmarked | Cannot be bookmarked |
ServletConfig | ServletContext | |
---|---|---|
1. | Parameters specified for a specific servlet. | Parameters specified for the entire application. |
2. | The object created during the initialization process. | The object created during web application deployment. |
3. | Available as long as the servlet is executing. | Available as long as the application is executing. |
Session tracking is a technique that servlets use to maintain a record of a series of requests originating from the same user or the same browser across a period of time. These sessions are shared between the servlets that are accessed by a client.
There are 4 techniques to track sessions:
- Cookies
- Hidden Fields.
- URL Rewriting.
- Session Tracking API.
Sessions | Cookies | |
---|---|---|
1. | Server-side files. | Client-side files. |
2. | Stores unlimited content in user's browser. | Stores limited content of limited users. |
3. | Data is not easy to modify. | Data is easy to modify. |
A filter is an object that filters tasks that are either request to a resource, or response from a resource, or both. Filtering is performed in doFilter method. Filters get configured in deployment descriptor of your application.
RequestDispatcher interface defines an object that can dispatch the request to resources like HTML, JSP, and Servlet, on the server. This interface provides two methods
forward (ServletRequest request, ServletResponse response) will forward a request from Servlet to another resource such as a Servlet, JSP, or HTML, on the server.
Include (ServletRequest request, ServletResponse response) will include the content of a resource such as Servlet, JSP, or HTML, in the response.
An Quick Overview of Servlet
In Java, servlets generate dynamic HTML content and respond with excel, XML, pdf, and JSON and other formats. Initially, servlets were meant for creating HTML content in applications, but now they are mostly used at the controller layers.
Our jsp servlet interview questions for experienced professionals is a huge hit amongst potential candidates looking for new jobs. Have you read them yet?
Development History of Servlet
Servlet 1.0 was released on June 1997.
Latest Version
Latest Version: Servlet 4.0 is the newest version that was released on September 2017 on the Java EE 8 platform. You should read in-depth about different versions and their features if you are preparing for tricky jsp and servlet interview questions .