React js interview questions and Answers
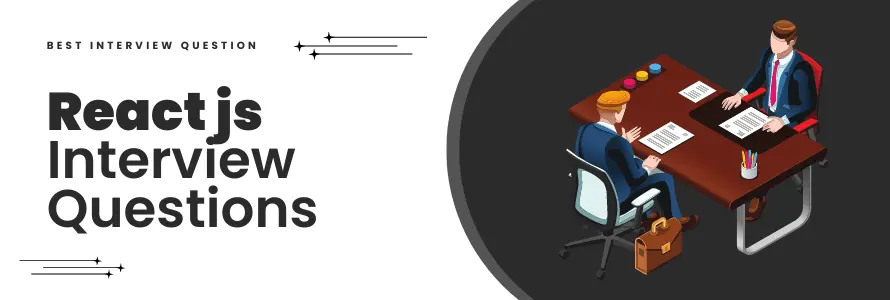
ReactJS is a JavaScript library for building reusable User interface components. It is a template-based language combined with different functions that support the HTML. Our recently updated React Interview Questions can help you crack your next interview. This means the result of any React's operation is an HTML code. You can use ReactJS to build all kinds of single-page applications.
Short Questions About React | |
---|---|
What is the latest version of React? | 18.0, released on March 29, 2022. |
When was React first released? | 29th May 2013 |
React JS is Created By | Jordan Walke |
License of ReactJS | MIT License |
React Developer Interview Questions
Real DOM | Virtual DOM |
---|---|
The update process is very slow in this. | The update process is much faster here. |
It can directly update the HTML. | It can't directly update the HTML. |
It creates a new DOM if the element is updated. | It updates the JSX if there is any update on an element. |
DOM manipulation related to a Real DOM is very complicated. | DOM Manipulation related to a Virtual DOM is effortless. |
There is a lot of memory wastage in a Real DOM. | There are no cases of memory wastage in a Virtual DOM. |
One should never update the state directly because of the following reasons:
- If you update it directly, calling the setState() afterward may just replace the update you made.
- When you directly update the state, it does not change this.state immediately. Instead, it creates a pending state transition, and accessing it after calling this method will only return the present value.
- You will lose control of the state across all components.
Note: Out of all the react questions, this is one that actually helps the interviewer to judge your level of understanding in this subject. Read the answer carefully to understand it properly.
To call a function inside a render in React, use the following code:
import React, { Component, PropTypes } from 'react';
export default class PatientTable extends Component {
constructor(props) {
super(props);
this.state = {
checking:false
};
this.renderIcon = this.renderIcon.bind(this);
}
renderIcon(){
console.log("came here")
return(
<div>Function called</div>
)
}
render() {
return (
<div className="patient-container">
{this.renderIcon}
</div>
);
}
}
Props stand for Properties in React. They are read-only components that must be kept pure. These components are passed down from the parent to the child component throughout the app. A child component cannot send the prop back to the parent component. This can help in maintaining the unidirectional data flow and is used to render the dynamically generated data.
The latest release of React is 16.6.9 and here are its newest features:
- Measuring performance using
- Improved Asynchronous act() for testing
- No more crashing when calling findDOMNode() inside a tree
- Efficient handling of infinite loops caused due to the setState inside of useEffect has also been fixed
JSX stands for JavaScript XML. It is a file type used by React that utilizes the expressiveness of JavaScript along with HTML. This makes the HTML file easy to understand. This file makes applications robust and boosts their performance.
render(){
return(
<div> <h1> Welcome To Best Interview Question!!</h1> </div>
);
}
A Controlled Component is one that takes the current value through the props and notifies of any changes through callbacks such as onChange.
Example:
<input type="text" value={value} onChange={handleChange} />
An uncontrolled component is one storing its own state internally, and you can query the DOM via a ref to find its current value as and when needed. Uncontrolled components are a bit closer to traditional HTML.
Example:
<input type="text" defaultValue="foo" ref={inputRef} />
Note: React is a widely used open-source library used for building interactive user interfaces. It is a web platform licensed under MIT. This is one of the very popular react interview questions.
Basically Function Based Component are the component which are made by JavaScript Functions.It takes props as argument and return JSX.
Import React from ‘react’;
const XYZ = (props ) => {
<div>
<h1>React Interview Questions</h1>
</div>
}
export default XYZ;
React | React Native |
---|---|
Released in the year 2013. | Released in the year 2015. |
It can be executed on multiple platforms. | It is not platform-independent. It will need some extra effort to work on different platforms. |
Generally used for developing web applications. | Generally used for developing mobile applications. |
Here, Virtual DOMs are used to render browser code. | Here, an in-built API is used to render code for mobile applications. |
It uses the JS library and CSS for animations. | It has its own in-built animation libraries. |
It uses the React Router to navigate through web pages. | It has an in-built navigator to navigate through mobile application pages. |
React Component | React Element |
---|---|
It is a simple function or class used for accepting input and returning a React element | It is a simple object used for describing a DOM node and the attributes or properties as per your choice. |
The React Component has to keep the references to its DOM nodes and also to the instances of the child components. | The React Element is an immutable description object, and in this, you cannot apply any methods. |
Top 20 React.js Interview Questions
Here you will find the list of Top React interview questions, which were written under the supervision of industry experts. These are the most common questions and usually asked in every interview for the role of the React Developers.
- What is HOC?
- What are the difference between Rest and Spread Operator?
- What are the key benefits of using Arrow functions?
- What is Pure Component?
- What are Controlled and Uncontrolled components with example?
- What is Refs?
- What is the difference between class based component & function based components?
- How to work React?
- Why virtual Dom is faster than real dom?
- How does virtual Dom work in react?
- What are the difference between null & undefined?
Conclusion
Now that you have read all the react questions, it is time for you to go towards the next level. Applying for a developer position would require you to actually code and not just give answers to theoretical questions.
That’s why you should enhance your skills by complementing the above react developer interview questions with a practical mindset. Start coding small stuff, get your concepts crystal clear. It’s all about how you can apply your knowledge in different projects. Code and code, that’s the secret to winning the dream job!