Python Interview Questions and Answers
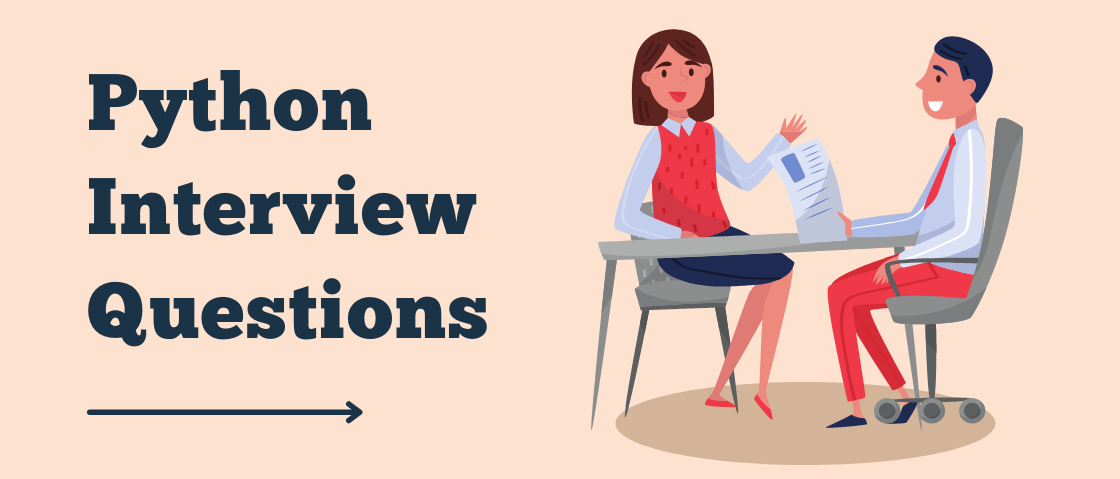
A high-level, interactive, and object-oriented scripting language, Python is a highly readable language that makes it ideal for beginner-level programmers. Here we can help you to prepare for the best Python interview questions. It uses English keywords and has fewer syntactical constructions as compared to other languages. Similar to PERL and PHP, Python is processed by the interpreter at runtime. Python supports the Object-Oriented style of programming, which encapsulates code within objects.
Python can be used for developing Websites, Web Apps, and Desktop GUI Applications. Here is a list of the most frequently asked Python Programming Interview Questions to learn more.
Quick Questions about Python | |
---|---|
What is the latest version of Python? | 3.8.3 and released on May 13, 2020. |
Who has invented Python? | Guido van Rossum |
What language does Python use? | C languages |
License | Python releases have also been GPL-compatible. |
Did you know, Python is also referred to as a “batteries included” language due to its in-depth and comprehensive standard library. Our Questions on Python have been selected from a plethora of queries to help you gain valuable insights and boost your career as a Python Developer.
Most Frequently Asked Python coding interview questions
In Python, a shallow copy essentially means building a new collection of objects and then referencing it with the child objects found in the original group of the object.
Note: Did you know, Python is also referred to as a “batteries included” language due to its comprehensive standard library. Our Python Interview Questions have been selected from a plethora of queries to help you gain valuable insights and boost your career as a Python Experts.
To create a named tuple in Python, follow these steps:
- Import the namedtuple class from the collections module.
- Now, the constructor shall take the name of the named tuple and a string containing the names of the field, separated by whitespace.
- The above action shall return a new namedtuple class for the specified fields.
- To use the new namedtuple, call the new class with all the values (in order) as parameters.
To send an email in Python, follow these steps along with the code:
import smtplib
sender = '[email protected]'
receivers = ['[email protected]']
message = """From: From Person <[email protected]>
To: To Person <[email protected]>
Subject: SMTP email test in Python
This is a test email message in Python.
"""
try:
smtpObj = smtplib.SMTP('localhost')
smtpObj.sendmail(sender, receivers, message)
print "Successfully sent the email"
except SMTPException:
print "Error display: Python is unable to send an email"
- Similar to PERL and PHP, Python is processed by the interpreter at runtime. Python supports the Object-Oriented style of programming, which encapsulates code within objects.
- Derived from other languages, such as ABC, C, C++, Modula-3, SmallTalk, Algol-68, Unix shell, and other scripting languages.
- Python is copyrighted, and its source code is available under the GNU General Public License (GPL).
- Supports the development of many applications, from text processing to games.
- Works for scripting, embedded code, and compiled the code.
- Detailed