PHP Array Functions
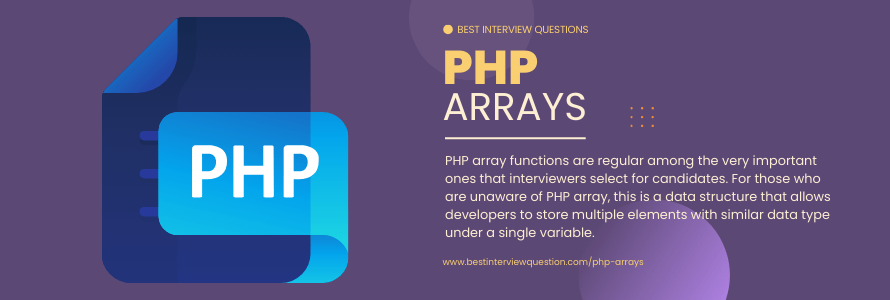
PHP array functions are regular among the very important ones that interviewers select for candidates. For those who are unaware of PHP array, this is a data structure that allows developers to store multiple elements with similar data type under a single variable, which save developers the effort to create a different variable for every data. The PHP arrays functions are extremely helpful creating a list of elements of similar types, which developers will able to access using their key or index.
Types of arrays in PHP;
- Numeric or Indexed Arrays,
- Multidimensional Arrays
- Associative Arrays
Most Frequently Asked PHP Array Functions
The array_filter() method filters the values of an array the usage of a callback function. This method passes each value of the input array to the callback function. If this callback function returns true then the current value from the input is returned into the result array.
Syntax:
array_filter(array, callbackfunction, flag)
- array(Required)
- callbackfunction(Optional)
- flag(Optional)
function test_odd_number(int $var)
{
return($var & 1);
}
$array=array(1,3,2,3,4,5,6);
print_r(array_filter($array,"test_odd_number"));
There are various methods in PHP to get the first element of an array. Some of the techniques are the use of reset function, array_slice function, array_reverse, array_values, foreach loop, etc.
Suppose we have an array like
$arrayVar = array('best', 'interview', 'question', 'com');
1. With direct accessing the 0th index:
echo $arrayVar[0];
2. With the help of reset()
echo reset($arrayVar);
3. With the help of foreach loop
foreach($arrayVar as $val) {
echo $val;
break; // exit from loop
}
PHP supports three types of arrays:-
- Indexed Array
- Associative array
- Multi-dimensional Array
1. array_pop()
It is used to delete or remove the last element of an array.
Example
$a=array("blue","black","skyblue");
array_pop($a);
OUTPUT : Array ( [0] => blue[1] => black)
2. array_push()
It is used to Insert one or more elements to the end of an array.
Example
$a=array("apple","banana"); array_push($a,"mango","pineapple");
OUTPUT : Array ( [0] => apple[1] => banana[2] => mango[3] => pineapple)
array_combine()
array_combine()
: It is used to create a new array by using the key of one array as keys and using the value of another array as values. The most important thing is using array_combine() that, number of values in both arrays should be same.
$name = array("best","interview","question");
$index = array("1","2","3");
$result = array_combine($name,$index);
array_merge()
array_merge()
: It merges one or more than one array such that the value of one array appended at the end of first array and if the arrays have same strings key then the later value overrides the previous value for that key .
$name = array("best","interview","question");
$index = array("1","2","3");
$result = array_merge($name,$index);
It is an inbuilt function in PHP. It is one of the most simple functions that is used to count all the values inside an array. In other words we can say that it is used to calculate the frequency of all of the elements of an array.
$array = array("B","Cat","Dog","B","Dog","Dog","Cat");
print_r(array_count_values($array));
// OUTPUT : Array ( [B] => 2 [Cat] => 2 [Dog] => 3 )
We can use the count() or sizeof() function to get the number of elements in an array.
$array1 = array("1","4","3");
echo count($array1);
OUTPUT : 3
To check key in the array, we can use array_key_exists().
$item=array("name"=>"umesh","class"=>"mca");
if (array_key_exists("name",$item))
{
echo "Key is exists";
}
else
{
echo "Key does not exist!";
}
$originalArray = array( 'ram', 'sita', 'luxman', 'hanuman', 'ravan' );
$newArray = array( 'kansh' );
array_splice( $originalArray, 3, 0, $newArray);
// OUTPUT is ram sita luxman hanuman kansh ravan
- sort() - It is used to sort an array in ascending order
- rsort() - It is used to sort an array in descending order
- asort() - It is used to sort an associative array in ascending order, according to the value
- ksort() - It is used to sort an associative array in ascending order, according to the key
- arsort() - It is used to sort an associative array in descending order, according to the value
- krsort() - It is used to sort an associative array in descending order, according to the key
Arrays help developers store multiple elements within a single variable that can be accessed using a key or an index. We can also combine it with for each statement to a quick loop through an array with the use of very little code. While web application development, we can store tabular data in a pair of nested arrays to achieve better performance and utility. PHP arrays functions also have various functions such as merge, sort, split and intersect which makes it easy for us to efficiently manipulate them. With the inefficiency of strong typing and OOP-object iteration support in PHP, arrays are the most preferable data structure to store and manipulate data. We will further continue here with top PHP Array interview questions picked by industry leaders with their suggested answers for your practice.