JQuery interview questions and Answers
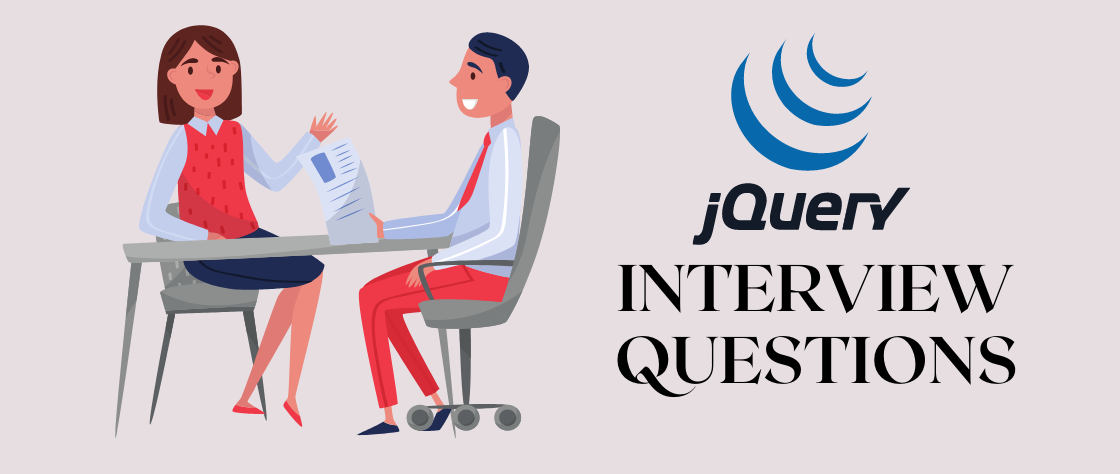
jQuery is a feature-rich, lightweight, and fast JavaScript library, which makes HTML documents, event handling, Ajax, and animation easier with a simple API that works across almost all browsers. These jQuery interview questions will enhance your skills and help you to write in a mainstream JavaScript library, used by major players. The critical purpose of jQuery is to make using JavaScript more convenient on your website. A great combination of extensibility and flexibility, jQuery takes a lot of general tasks that require multiple lines of JavaScript code and wraps them in a manner that you can use with a single line of code.
Most Frequently Asked JQuery interview questions
In the standard URL- encoded notation jQuery serialize() is used to create the text strings. It also forms several other controls.
$("button").click(function(){
$("div").text($("form").serialize());
});
Alert()- it is a pop up that is intended for the user to click the OK button and vanish once you click.
Confirm ()- it is a wider choice and gives 2 options to the user to choose. It is basically like an if-else statement.
Display message with alert() and confirm()
alert('Are you sure to delete this item?');
confirm("Are you sure to delete this item?");
Onclick- it is a mouse event and occurs when the user clicks the left button of the mouse.
Onsubmit- it is a form event and occurs when a user is trying to submit the form. When form validation is used, then this is considered.
$("p").click(function(){
alert("You clicked here");
});
$("form").submit(function(){
alert("You form submitted");
});
In order to concatenate two strings in jQuery concatenate the strings using + consternation operator. You have to follow a sequence of codes soon after it. Once it is done, the strings get concatenate. There are some other alternatives as well.
var a = 'Best Interview';
var b = 'Question';
var result = a + b;
// output
Best Interview Question
You can check it in various ways.
if ($('#element').is(':empty')){
//write your code here
}
OR
if ( $('#element').text().length == 0 ) {
// length is 0
}
remove() | detach() | empty() |
---|---|---|
through it matched elements can be removed completely from the DOM | it is more like with remove function, but it stores the data associated with elements. | it removes all the child elements from the data. |
$.ajax()
- for async request$.ajaxsetup()
- to set default value$.ajaxTransport()
- for object transmission$.get()
- loads data$.ajaxPrefilter()
- handle custom solutions$.getJSON()
- loads JSON data
The latest version of jQuery is jQuery3.3
The text() method return text value inside an HTML element whereas HTML() method returns the entire HTML syntax.
The text() method is used to manipulate the value and the HTML() method is used to manage the HTML object or properties.
With the help of CSS() method, we can add or remove CSS properties to an HTML element.
Syntax
$(selector).css(property);
$("#divID").css("display", "block");
Development History of jQuery
jQuery was released by John Resig at the BarCamp NYC in January 2006. Currently, it is headed by Timmy Wilson and managed by a team of expert developers.
Latest Version: The most recent version is 3.5.0, which was released on 4th May 2020.
Advantages
- Easy to learn and intuitive
- Deals with many cross-browser bugs
- Clean and simple syntax
- Open source library
- Highly extensible
These jQuery interview questions will help you prepare for your upcoming job interview as well and fast-track your career, whether you are a fresher or an experienced Jquery developer.